With Lambda and AWS API Gateway we are able to run a typical ASP.NET Web API application by replacing the web server as so: AWS Serverless Web API. The AWS Toolkit for Visual Studio provides a project template for Web API on Lambda. The whole Web API project can be deployed into S3. Details of this can be found here. With Visual Studio, you can install AWS's AWS.NET Mock Lambda Test Tool. Use the following command in the same directory as your Solution file: dotnet tool install -g Amazon.Lambda.TestTool-2.1 Visual Studio automatically adds a Debug configuration so you can debug the project just like you would any other.NET app. Visual Studio 2019 Community; AWS Toolkit for Visual Studio; Open your Visual Studio and follow the path. And now we are ready to deploy our API to AWS Lambda. Back within the terminal in Visual Studio Online run aws configure and copy the Access Key ID and Secret Access Key into the prompts. This step took me about 5 minutes. So, we are ready to start building the lambda in about 10 minutes. Make a Python Lambda and Publish. The goal of this post is not really the Lambda more just to get it all set up.
Full source code available here.
A few months ago AWS released a feature allowing Lambdas to run container images, for larger applications this is easier to work with than a zip file or set of layers, it also lets you move your already containerized apps to Lambda with a small effort.
I was interested to see if I could get a .NET 5 “Hello World” application running in this manner. There is a blog on the AWS site explaining how to do this with Visual Studio and the AWS Toolkit, but primarily use VS Code so I could not leverage those tools, and it would be fun to figure out.
UPDATE 1
When I published this blog post I was unaware of a pre-made .NET 5 Docker image for AWS Lambda so I built my own following instructions found on an AWS GitHub repo. Those instructions are below.But it is much easier to use the AWS provided image available here - https://gallery.ecr.aws/lambda/dotnet and use public.ecr.aws/lambda/dotnet:5.0
.
UPDATE 2
The AWS Lambda image above seems to have the full .NET 5 ASP.NET Runtime as opposed to the .NET 5 Runtime, if all you are running in the container is a library, then having the full .NET 5 ASP.NET Runtime will be larger than you need. As of now, I don’t see an image on the AWS ECR page for the .NET 5 Runtime. But you can build it your self following the below instructions, with one change, on line 49 of this file https://github.com/aws/aws-lambda-dotnet/blob/087590ce99274e16e26d37e1dfd73b0b71d1230a/LambdaRuntimeDockerfiles/dotnet5/Dockerfile, change the linked tar.gz
to the appropriate file in here https://versionsof.net/core/5.0/.
Building your own base image (optional)
You can’t use just any docker image for a .NET 5 application, it has to have special Lambda and AWS components included, here is how to build your own, if you want to, from the AWS code on GitHub.
- clone https://github.com/aws/aws-lambda-dotnet
- build this image https://github.com/aws/aws-lambda-dotnet/blob/master/LambdaRuntimeDockerfiles/dotnet5/Dockerfile
- reference this built image in the Dockerfile as the base
But it is much easier to use the one image AWS provides - https://gallery.ecr.aws/lambda/dotnetNow that you have a base image, let’s move to the application.
Building the hello world container
Create a new .NET Lambda using -
This crates a simple library that converts an input string to uppercase.If you wanted to, you could build this, zip it and deploy it to a Lambda as I showed in part 1 of this series.
But I want to put this in a container.
You need Docker for the rest of this, there are plenty of tutorials out there including one from my friend Steve Gordon.
In the source code directory add a file named Dockerfile
with the below content. Note that it’s using the image I put on Docker Hub.
Everything is in place, build the image.
Over in AWS we need a repository to store the image.
Go to the Elastic Container Registry.
Create a repository.
Navigate into the repository and you will see a button labeled “View push commands”. Follow those instructions to upload the container image to repository.
That’s everything done here, over to the Lambda.
The Lambda
This is similar to what I showed in part one, but this time the function will be based on a container image.
Create a new Lambda function, select Container image from the options.
Give the function a name.
Hit Browse images, and select the container image from the repository created above.
It will take a few moments for AWS to create the function. Once it is up, open the test tool in the top right of the screen and click “Configure test events”.
Set the event name, and replace the body with this - “hello world”.
Hit the “Test” button in the top right.
You should see something like - “Execution result: succeeded(logs)”. Expand the Details and you will see “HELLO WORLD” and bunch of other information about the execution of the Lambda.
That is a .NET 5 library running inside container, running inside a Lambda, not bad!
But what if you could get Kestrel up and running and map API Gateway requests to it, that would be fun…and that’s in the next post of this series.
Full source code available here.
Related
So mostly for entertainment sake and to see how easy it would be, I wondered if I could create a Python-based lambda in AWS using GitHub and Visual Studio Online. So, let’s see how this goes and how long it takes …
Pre-Requisites
For this little experiment, you will need:
- A GitHub account
- An Azure account
- An AWS account
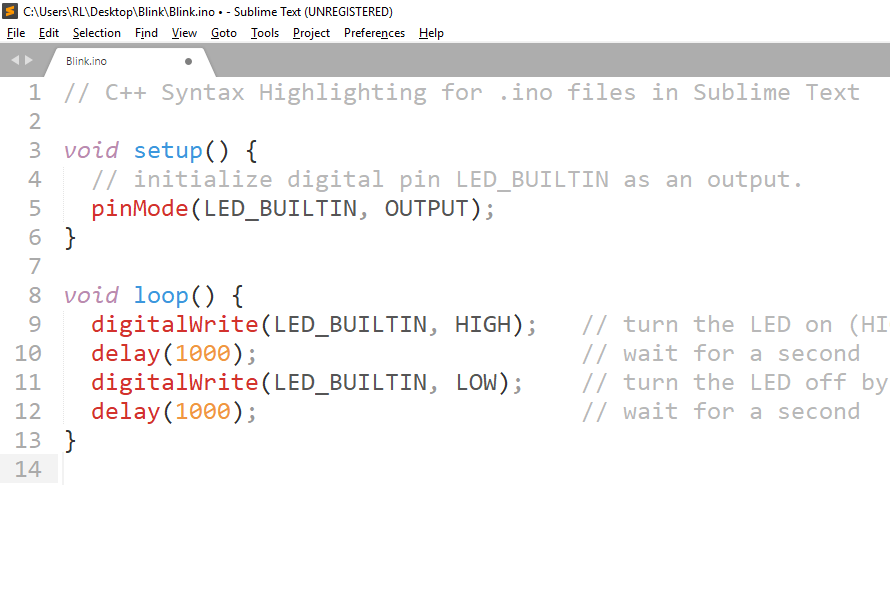
Set Up the Development Environment
Aws Lambda Visual Studio Code
Let’s create an empty repository in GitHub as we need somewhere to keep code! Go to https://github.com/new and create a new repository, I called mine PythonLambda
. I made it public and with a README (so not empty to start).
Next hop over to Visual Studio Online and click get started. You will then need to log in using your Azure account. Then click Create Environment. Enter in a name (I chose the same as the GitHub project) and then paste the URL for the GitHub repo into the Git repository. Click create and wait for it to be available.
Next, connect to the environment. A window that looks remarkably like Visual Studio Code will appear; I chose to install support for Python. This took virtually no time whatsoever. Next hit Ctrl-'
to open the terminal windows. Let’s check for python by running python --version
:
On to installing the AWS CLI. Simply run the command pip3 install awscli --upgrade --user
. Once this completes you can run aws --version
:
So now we have a working development environment. Took about 3 minutes to set this up. We have a git repository, a copy of Visual Studio Code set up to write python code and the AWS CLI.
Logging into GitHub and AWS
Next, we can edit the README and check we can push back to GitHub as a new branch. Change the README file and save it. You can then go to Source Control tab (press Ctrl-Shift-G
), commit the files and push to GitHub. It will pop up a window asking you to authorise microsoft-vs
to access your GitHub account. After that, it will push the code to GitHub.
Next head to the AWS IAM console and log in. Ideally, you will create a new user but for the sake of simplicity, I will just create a new access key. Go to the Users
link, find your user, go to Security Credentials
and select Create Access Key
. Back within the terminal in Visual Studio Online run aws configure
and copy the Access Key ID and Secret Access Key into the prompts.
This step took me about 5 minutes. So, we are ready to start building the lambda in about 10 minutes.
Make a Python Lambda and Publish
The goal of this post is not really the Lambda more just to get it all set up. The code below is a really trivial function. Create a new file called lambda.py
and add the new content:
Aws Lambda Visual Studio 2017
This is a very simple function which will log whatever is passed to it and return the servers current date.
The bare minimum we need to do for a lambda to run is to create an IAM role and policy, and then we can publish a function. Run the following within the terminal:
This will create the Role and Policy for the lambda to use. Finally, lets publish the first version of the lambda. In the terminal, run:
Finally, we will set up a build task to publish this to AWS. Create a new folder called .vscode
and add a new file called tasks.json
. Add the following content:
Now if you hit Ctrl-Shift-B
within the editor, it will zip and deploy the python code as it stands to AWS.
Wrapping Up
Visual Studio Online is amazing. You can get a development environment up and running in minutes. It works anywhere and can easily be hooked into whatever you like. While this post has only created a very basic set up, it is hopefully enough to show you how you could do everything from the comfort of your own browser and in very little time.
Aws Lambda Visual Studio C#
This post was also published on my own personal blog - jdunkerley.co.uk